Do you want to use conditional display rules in Thrive Architect? You can use this to decide when you want to show what depending on the trigger you’ve set.
This article will show you how you can create them.
Please note that creating custom rules is an action that should be performed by developers or more tech-savvy persons, as it does require technical knowledge. |
Example 1: You can add a new field, called “Page – Demo” with a subfield called “Title – Demo”. It will also add a field called “Number of comments – Demo” to the already existing “User” entity.
After activating the plugin, the new fields will be visible in the conditional display pop-up of Thrive Architect:
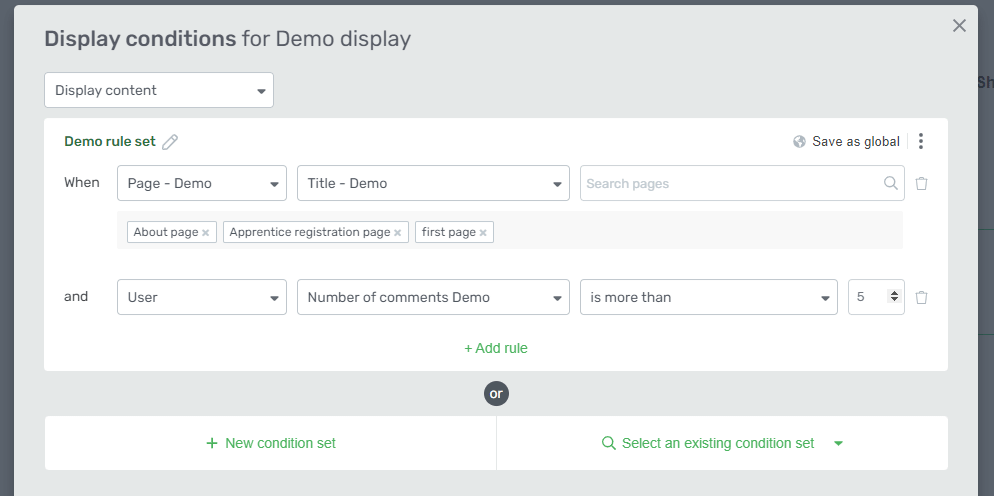
Note: You can find this demo example on GitHub, here. |
Example 2: we’ll show how to create a new rule that is conditioned by whether the user has WP Fusion tags assigned to them or not.
Here are the steps to be taken in order to create a new rule:
- Defining the Entity Class
- Extending the Entity Class and Defining Specific Properties to Describe Your Subject
- Registering Entity Class Using the Function
- Creating an Entity Field That Will Be Used to Fetch the Specific Entity Data Which the Condition Will Be Tested On
Defining the Entity Class
Firstly, an entity class will have to be defined. This should describe the subject/entity, as well as its properties.
class User extends TCBConditionalDisplayEntity
/**
* @return string
*/
Extending the Entity Class and Defining Specific Properties to Describe Your Subject
The get_key
function defines a unique identification key for the entity:
public static function get_key() {
return 'user_data';
}
The get_label
function defines the name of the entity that will be visible to the users.
This will appear inside the visual editor.
public static function get_label() {
return esc_html__( 'User', 'thrive-cb' );
}
The create_object
property, upon running the condition logic, will create the entity object containing all the data representing individual fields.
public function create_object( $param ) {
$user_id = get_current_user_id();
return get_userdata( $user_id );
}
/**
* Determines the display order in the modal entity select
*
* @return int
*/
public static function get_display_order() {
return 0;
}
Registering Entity Class Using the Function
tve_register_condition_entity
function tve_register_condition_entity( $entity ) {
TCBConditionalDisplayEntity::register( $entity );
}
Use the entity class as a parameter.
As a result, once you put everything together, the code snippet will look like this:
class User extends TCBConditionalDisplayEntity {
/**
* @return string
*/
public static function get_key() {
return 'user_data';
}
public static function get_label() {
return esc_html__( 'User', 'thrive-cb' );
}
public function create_object( $param ) {
$user_id = get_current_user_id();
return get_userdata( $user_id );
}
/**
* Determines the display order in the modal entity select
*
* @return int
*/
public static function get_display_order() {
return 0;
}
Creating an Entity Field That Will Be Used to Fetch the Specific Entity Data Which the Condition Will Be Tested On
Example (in the visual editor):
Extend the field class and define specific properties to describe your field
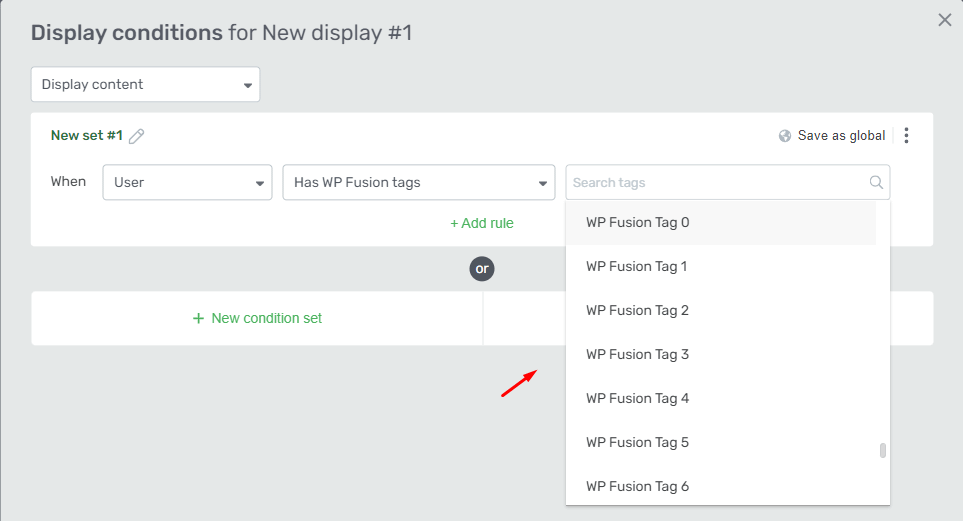
class User_Wpfusion_Tags extends TCBConditionalDisplayField {
/**
* @return string
*/
The get_key
function defines a unique identification key for the field.
public static function get_key() {
return 'user_wpfusion_tags';
}
The get_label
function defines the name of the field that will be visible to the users.
This will appear inside the visual editor.
public static function get_label() {
return __( 'Has WP Fusion tags', TVE_DASH_TRANSLATE_DOMAIN );
}
The get_conditions
property describes the type of condition that the field data represents.
A list of possible operator/condition keys to use in the get_conditions
from a field, along with an example of an entity + field group where they are currently used:
- autocomplete ( User -> Username )
- autocomplete_hidden ( User -> Product_Access )
- checkbox ( User -> Role )
- date ( Time -> Current date )
- date_and_time ( Can be used when you don’t want to include date intervals – see below )
- date_and_time_with_intervals ( User -> Last logged in )
- dropdown ( Post -> Post status )
- number_comparison ( Post -> number of comments )
- number_equals ( Time -> Day of month )
- string_comparison ( Request -> Cookie )
- time ( Time -> Time field )
- url_comparison ( Referral -> Url )
public static function get_conditions() {
return [ 'autocomplete' ];
}
/**
* @return string
*/
public static function get_entity() {
return 'user_data';
}
The get_conditions
type can be one of the registered types of conditions that extend the condition class, or you can create your own and register it, using the tve_register_condition
function:
/**
* Register a new condition
*
* @param TCBConditionalDisplayConditionstring $condition
*/
function tve_register_condition( $condition ) {
TCBConditionalDisplayCondition:: register ( $condition 0;
}
Use the condition class as a parameter.
The get_value
property has to be defined. This will fetch the value from the entity upon running the condition logic.
public function get_value( $user_data ) {
$tags = wp_fusion()->user->get_tags( $user_data->ID );
return empty( $tags ) ? '' : $tags;
}
public static function get_options( $selected_values = [], $searched_keyword = '' ) {
$levels = [];
The $user_data parameter will contain the entity data object.
For multiple-choice Fields:
The property get_options
has to be defined and the values/label pairs of options have to be provided.
public static function get_options( $selected_values = [], $searched_keyword = '' ) {
$levels = [];
foreach ( wp_fusion()->settings->get_available_tags_flat() as $tag ) {
if ( static::filter_options( $tag, $tag, $selected_values, $searched_keyword ) ) {
$levels[] = [
'value' => (string) $tag,
'label' => $tag,
];
}
}
return $levels;
}
$selected_values will contain the values already chosen;
$searched_keyword will be used to filter dropdown results;
As a result, once you put everything together, the code snippet looks like this:
class User_Wpfusion_Tags extends TCBConditionalDisplayField {
/**
* @return string
*/
public static function get_key() {
return 'user_wpfusion_tags';
}
public static function get_label() {
return __( 'Has WP Fusion tags', TVE_DASH_TRANSLATE_DOMAIN );
}
public static function get_conditions() {
return [ 'autocomplete' ];
}
/**
* @return string
*/
public static function get_entity() {
return 'user_data';
}
public function get_value( $user_data ) {
$tags = wp_fusion()->user->get_tags( $user_data->ID );
return empty( $tags ) ? '' : $tags;
}
public static function get_options( $selected_values = [], $searched_keyword = '' ) {
$levels = [];
foreach ( wp_fusion()->settings->get_available_tags_flat() as $tag ) {
if ( static::filter_options( $tag, $tag, $selected_values, $searched_keyword ) ) {
$levels[] = [
'value' => (string) $tag,
'label' => $tag,
];
}
}
return $levels;
}
public static function get_autocomplete_placeholder() {
return __( 'Search tags', TVE_DASH_TRANSLATE_DOMAIN );
}
}
Registering the Field Class Using the Function
tve_register_condition_field
/**
*Register a new condition field
*
*@param TCBConditionalDisplayField|string $field
*/
function tve_register_condition_field( $field ) {
TCBConditionalDisplayField::register( $field );
}